Pythonプログラムを勉強する上で、NW機器のログ自動取得ツールを作成しています。
ログを取得する機器は主にSSHアクセスが可能な以下機器を想定しています。
- NW機器全般:Cisco、juniperなど
- サーバー:linux系サーバー
- 仮想基盤:VMware(ESXi)
また、やるならGUI画面から操作をしたいと言うこともあり、デスクトップアプリツール「tkinter」をインストールして作成しています。
まずは試作第1号として、作成プログラムと試作品を提供します。
【Python】NW機器ログ取得ツール試作機作成(Ver.01)
まず、このツール作成の目的ですが、毎回ターミナルツールを立ち上げてログを取得するのがめんどくさいのを実行ボタン一つで一気にコマンド実行とファイル取得をしたいと言うことから作成しました。
また、WindowsでもMacでもどちらのOSでも利用できるようにしています。
ファイル実行後の画面となります。
※Macで立ち上げた画面
ファイルの立ち上げはexeファイルで実施すると以下の画面が表示されます。
SSHアクセスさせてログを取得したいコマンドを入力し「実行する」を押します。
- host:対象機器のIPアドレス
- name:ログインID
- password:パスワード
- command:実行したいコマンド
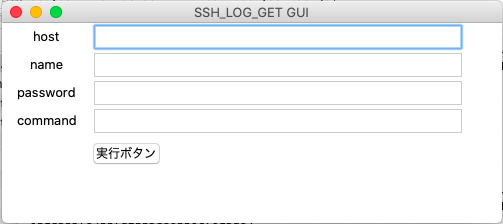
実行をした後、成功すれば同じフォルダに「file。log」が作成されます。
中身を見ると、実施したコマンドの出力結果が表示されます。
今回は、「ls」コマンドを実行しました。
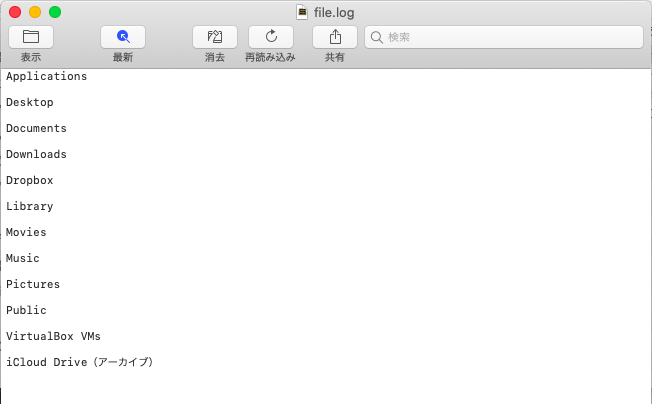
ログ取得ツールプログラム情報共有
今回のログ取得ツールのプログラムですが、私が作成した情報を共有します。
実際にいろんな方に作っていただき、色々と改善点など情報をいただき、どんどんバージョンアップし、便利なツールを作っていきたいと思います。
ぜひ、使ってください。
また、Pythonのインストールやテキストエディタなどの設定手順はこのサイトで紹介していますので、参考にして立ち上げてください。
以下が、本ツールのプログラムとなります。
#!python3.6 import tkinter import paramiko import datetime import pandas as pd import re from tkinter import messagebox #ボタンがクリックされたら実行 def button_click(): input_host_value = input_name.get() input_name_value = input_address.get() input_address_value = input_phone.get() input_command_value = input_command.get() client = paramiko.SSHClient() client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) client.connect(input_host_value, username=input_name_value, password=input_address_value) stdin, stdout, stderr = client.exec_command(input_command_value) stdin.write(input_command_value) for o in stdout: with open('file.csv', 'a', newline='') as f: print(o, end='', file = f) for e in stderr: print(e) client.close() root.quit() print('コンフィグ取得 > csvファイル保存完了') # tkinter_ウインドウの作成 root = tkinter.Tk() root.title("SSH_LOG_GET GUI") root.geometry("350x120") # host/IPaddress input_name_label = tkinter.Label(text="host") input_name_label.grid(row=1, column=1, padx=10,) # host/IPaddress入力欄の作成 input_name = tkinter.Entry(width=40) input_name.grid(row=1, column=2) # userID input_address_label = tkinter.Label(text="name") input_address_label.grid(row=2, column=1, padx=10,) # userID入力欄の作成 input_address = tkinter.Entry(width=40) input_address.grid(row=2, column=2) # パスワード input_phone_label = tkinter.Label(text="password") input_phone_label.grid(row=3, column=1, padx=10,) # パスワード入力欄の作成 input_phone = tkinter.Entry(width=40) input_phone.grid(row=3, column=2) # コマンド input_command_label = tkinter.Label(text="command") input_command_label.grid(row=4, column=1, padx=10,) # コマンド入力欄の作成 input_command = tkinter.Entry(width=40) input_command.grid(row=4, column=2) #ボタンの作成 button = tkinter.Button(text="実行ボタン",command=button_click) button.place(x=10, y=80) #ウインドウの描画 root.mainloop() # 出力結果のスペースをカンマ区切りに変換 with open('file.csv', 'r', newline='') as file, \ open('file_out.csv', 'w', newline='') as fileout: text = re.sub(r'\s* ', ',', file.read()) print(text, file = fileout) print('置換完了') # CSVファイルの読み込み col_names = ['c{0:02d}'.format(i) for i in range(100)] #data = pd.read_csv('file_out.csv', encoding="shift-jis", names = col_names) data = pd.read_csv('file_out.csv', encoding='cp932', names = col_names) # Excel形式で出力 data.to_excel('excel-data.xlsx', encoding='utf-8') print('CSV > Excel変換完了')
注意事項
上記プログラムを実行の際、以下のようなエラーが発生する場合があります。
以下は、対象のモジュールがインストールされていないためのエラーですので該当のモジュールをインストールしておきましょう。
$ python3 ssh-test.pyw Traceback (most recent call last): File "ssh-test.pyw", line 3, in <module> import paramiko ModuleNotFoundError: No module named 'paramiko'
今回、使用したモジュールは以下となります。
- paramiko:SSHモジュール
- tkinter:デスクトップツール(GUI)
$ pip3 install paramiko $ pip3 install tkinter
また、プログラムは上記で提供していますので実際に動かしてみて、ご意見をいただけると幸いです。
※あくまでテストツールなので、検証用として使ってください。
何か問題が起きたことによる保証はしません。
今後の課題
今後、このツールをより良いものにするために以下の課題が出てきました。
- 複数機器に対しSSHを実施し、一気にログ取得をしたい
- ファイル名をログイン先のホスト名にし、日付も付けたい
- 一度入力した情報を自動で記憶(save)したい
- 対象機器の種類とコマンド選択ができるようにしたい
今後もPythonを勉強しながらツールを作っていきたいと思います。
よろしくお願いします。
エンジニアのオンライン学習
ITエンジニアにおすすめの教材、オンラインスクールです。
無料からエンジニアの学習ができる教材などまとめているので参考にしてください。
おすすめオンライン教材 | |
自宅で学習ができるオンラインスクール | |
ITエンジニアの開発・検証・学習としてインターネット上で専用のサーバ(VPS)を利用しましょう!
実務経験はVPSで学べます。